
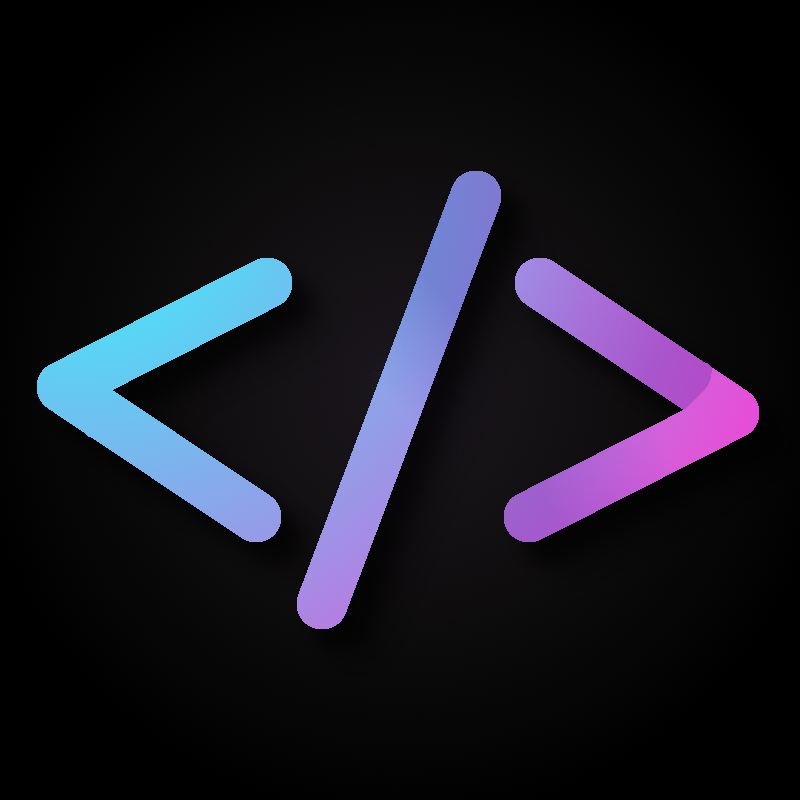
void foo() {
std::vector v = {0, 1, 2, 4};
const auto& ref = v[1];
add_missing_values(v);
std::cout << ref << "\n";
}
void add_missing_values(std::vector<int>& v) {
// ...
v.push_back(3);
}
Neither foo(), nor add_missing_values() looks suspicious. Nonetheless, if v.push_back(3)
requires v
to grow, then ref
becomes an invalid reference and std::cout << ref
becomes UB (use after free). In Rust this would not compiles.
It is order of magnitudes easier to have lifetime errors in C++ than in Rust (use after free, double free, data races, use before initialisation, …)
That’s why I did not said it was impossible, just order of magnitude harder to catch in C++ compared to Rust.
To have asan finding the bug, you need to have a valid unit test, that has a similar enough workload. Otherwise you may not see the bug with asan if the vector doesn’t grow (and thus
ref
would still be valid, not triggering UB), leading to a production-only bug.Asan is a wonderfull tool, but you can’t deny it’s much harder to use and much less reliable than just running your compiler.